PHP MySQLi Login Page Example
Here is an example of login page using PHP and MySQLi.
<?php // you have already learned about session session_start(); $conn = mysqli_connect('localhost', 'root', '', 'codescracker'); if(mysqli_connect_error()) { echo "<p>Error in connection to database.</p>"; exit(); } if($_SERVER["REQUEST_METHOD"] == "POST") { // checks whether logout button is clicked or not if(isset($_POST["logout"])) { // below code is used to destroy all the session session_destroy(); // below code is used to redirect users to codescracker.php page header("location: codescracker.php"); // below code is used to skip executing the remaining code // after this exit(); } $username = $password = ""; $username = $_POST["username"]; $username = filter_login_input($username); $password = $_POST["password"]; $password = filter_login_input($password); $qry = "select * from users where username='$username' and password='$password'"; $res = $conn->query($qry); if(mysqli_num_rows($res)>0) { $_SESSION['login'] = $username; } else { $loginCheck = "No"; } } function filter_login_input($loginData) { $loginData = trim($loginData); $loginData = stripslashes($loginData); $loginData = htmlspecialchars($loginData); return $loginData; } ?> <html> <head> <title>PHP and MySQLi Login Page Example</title> <script> function checkBeforeLogin() { if(document.loginForm.username.value=="") { alert("Enter Username"); document.loginForm.username.focus(); return false; } if(document.loginForm.password.value=="") { alert("Enter Your Password"); document.loginForm.password.focus(); return false; } return true; } </script> </head> <body> <?php if(isset($_SESSION['login'])) { echo "<p>You are successfully logged in.</p>"; echo "<p>Now you can access the admin page.</p>"; echo "<form method=\"post\">"; echo "<input type=\"submit\" name=\"logout\" value=\"LogOut\">"; echo "</form>"; exit(); } ?> <h2>LogIn</h2> <form name="loginForm" method="post" onSubmit="return checkBeforeLogin()"> <input name="username" type="text" placeholder="enter username" maxlength="40" required><br/> <input name="password" type="password" placeholder="enter password" maxlength="40" required><br/> <?php if(isset($loginCheck)) { echo "Invalid data<br/>"; } ?> <input type="submit" name="login" value="LogIn"> </form> </body> </html>
Save the above code in a file with name codescracker.php inside the directory C:\xampp\htdocs\ and run the above example code of login page just by opening any web browser and typing localhost/codescracker.php.
Here is the sample output produced by the above example of login page using PHP and MySQLi.
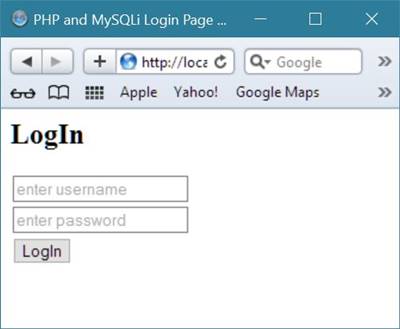
Now, let's first enter wrong data as shown in the following figure:
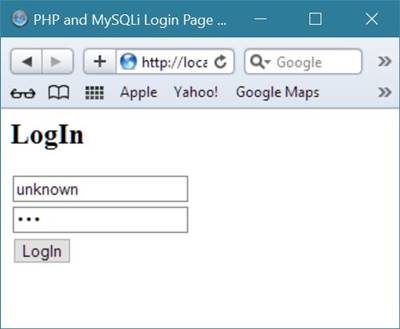
After entering the above wrong data (username and password that is not stored inside the database), click on the LogIn button, you will see the following output:
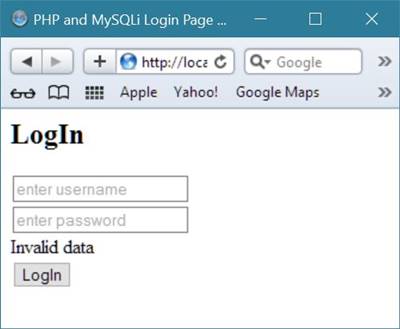
Now enter the right data this time, that is, codescracker as username and password both and click on the LogIn button. As username and password as codescracker is stored inside the database, therefore here is the output that will be produce in your browser:
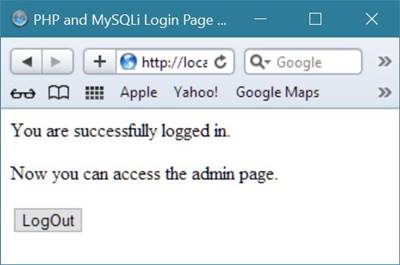
Now again type localhost/codescracker.php in your web browser, then the output will be same, that is, you are logged in, as we have used session here to keep the login information of the user.
If you want to logout from the page, then you must have to click on the LogOut button.
0 comments:
Post a Comment