We have dealt only with variables that store single values, called scalar variables. In this lesson, we will be covering arrays. Arrays are variables that store sets of values.
Lesson Goals
- Work with indexed arrays.
- Work with associative arrays.
- Work with two-dimensional arrays.
- Work with array-manipulation functions.
Indexed Arrays
Indexed arrays are similar to tables with a single column. An indexed array can contain zero or more elements. In PHP, like in many programming languages, the first element of an array is in the "zeroeth" position. An array with no elements has a zero length.
Initializing Arrays
Arrays are initialized with the
array()
function, which can take a list of comma-delimited values that become the elements in the new array. The following lines of code initializes a zero-length array and then adds four elements to the array.Syntax
$beatles = array(); $beatles[0] = 'John'; $beatles[1] = 'Paul'; $beatles[2] = 'George'; $beatles[3] = 'Ringo';
The first line above is actually optional as the second line will create the array if one does not already exist. However, it is a better coding practice to explicitly initialize the array. The
$beatles
array could also be created in a single line as follows.Syntax
$beatles = array('John','Paul','George','Ringo');
Appending to an Array
If you know how many elements are in an array, you can append to the array by specifying the index. For example, you could append to the
$beatles
array shown above as follows:Syntax
$beatles[4] = 'Nat';
However, sometimes you don't know how many elements are in an array. Although you can easily figure this out, doing so requires an extra step. PHP provides an easy way of appending to an array of any length. Simply leave out the index.
Syntax
$beatles[] = 'Nat';
Reading from Arrays
Reading from arrays is just a matter of pointing to a specific index or key.
Syntax
echo $beatles[2]; //outputs George to the browser
Looping through Arrays
The following code will loop through the entire
$beatles
array outputting each element to the browser.Syntax
foreach ($beatles as $beatle) { echo "$beatle<br>"; }
The above code snippets are combined in the following example.
Code Sample:
Arrays/Demos/IndexedArrays.php
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title>Indexed Arrays</title> </head> <body> <h1>Indexed Arrays</h1> <?php $beatles = array(); $beatles[0] = 'John'; $beatles[1] = 'Paul'; $beatles[2] = 'George'; $beatles[3] = 'Ringo'; echo $beatles[2]; //outputs George to the browser $beatles[] = 'Nat'; ?> <hr> <?php foreach ($beatles as $beatle) { echo "$beatle<br>"; } ?> </body> </html>
Working with Indexed Arrays
Duration: 10 to 15 minutes.
In this exercise, you will use arrays to create a table with a single column that lists all your favorite colors. As shown in the screenshot below, the background of each table row should be the same as the color named in the row.
See https://www.w3schools.com/colors/colors_names.asp for a list of color names.
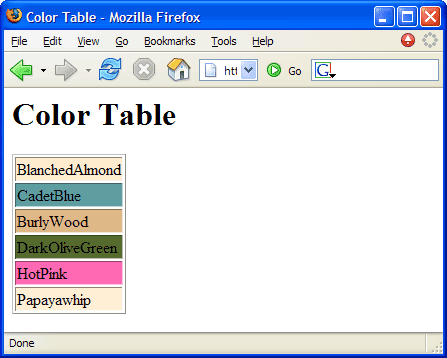
- Open Arrays/Exercises/ColorTable.php for editing.
- Create an array that holds your favorite colors.
- Inside of the open and close
<table>
tags, loop through the array outputting a table row for each element. - Test your solution in a browser.
Solution:
Arrays/Solutions/ColorTable.php
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title>Color Table</title> </head> <body> <h1>Color Table</h1> <?php $favColors = array(); $favColors[] = 'BlanchedAlmond'; $favColors[] = 'CadetBlue'; $favColors[] = 'BurlyWood'; $favColors[] = 'DarkOliveGreen'; $favColors[] = 'HotPink'; $favColors[] = 'Papayawhip'; ?> <table border="1"> <?php foreach ($favColors as $color) { echo "<tr style='background-color:$color'><td>$color</td></tr>"; } ?> </table> </body> </html>
Associative Arrays
Whereas indexed arrays are indexed numerically, associative arrays are indexed using names. For example, instead of Ringo being indexed as 3, he could be indexed as "drummer".
Initializing Associative Arrays
Like with indexed arrays, we can intialize a zero-length associative array and then add elements.
Syntax
$beatles = array(); $beatles['singer1'] = 'Paul'; $beatles['singer2'] = 'John'; $beatles['guitarist'] = 'George'; $beatles['drummer'] = 'Ringo';
Or the array could be created in a single line as follows.
Syntax
$beatles = array('singer1' => 'John', 'singer2' => 'Paul', 'guitarist' => 'George', 'drummer' => 'Ringo');
Reading from Associative Arrays
Reading from associative arrays is as simple as reading from indexed arrays.
Syntax
echo $beatles['drummer']; //outputs Ringo to the browser
Looping through Associative Arrays
The following code will loop through the entire
$beatles
array outputting each element and its key to the browser.Syntax
foreach ($beatles as $key => $beatle) { echo "<b>$key:</b> $beatle<br>"; }
The above code snippets are combined in the following example.
Code Sample:
Arrays/Demos/AssociativeArrays.php
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title>Associative Arrays</title> </head> <body> <h1>Associative Arrays</h1> <?php $beatles = array('singer1' => 'John', 'singer2' => 'Paul', 'guitarist' => 'George', 'drummer' => 'Ringo'); echo $beatles['drummer']; //outputs Ringo to the browser ?> <hr> <?php foreach ($beatles as $key => $beatle) { echo "<b>$key:</b> $beatle<br>"; } ?> </body> </html>
Superglobal Arrays
The superglobal arrays are associative arrays. The file below outputs all the contents of the superglobal arrays using
foreach
loops.Code Sample:
Arrays/Demos/SuperGlobals.php
<?php session_start(); ?> <!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title>Superglobal Arrays</title> </head> <body> <h1>Superglobal Arrays</h1> <h2>$_COOKIE</h2> <ol> <?php foreach ($_COOKIE as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_ENV</h2> <ol> <?php foreach ($_ENV as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_FILES</h2> <ol> <?php foreach ($_FILES as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_GET</h2> <ol> <?php foreach ($_GET as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_POST</h2> <ol> <?php foreach ($_POST as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_REQUEST</h2> <ol> <?php foreach ($_REQUEST as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_SESSION</h2> <ol> <?php foreach ($_SESSION as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> <hr> <h2>$_SERVER</h2> <ol> <?php foreach ($_SERVER as $key => $item) { echo "<li><b>$key:</b> $item<br></li>"; } ?> </ol> </body> </html>
Don't worry about the
session_start()
statement at the top. We'll cover that in detail later in the course.Working with Associative Arrays
Duration: 10 to 15 minutes.
In this exercise, you will use arrays to create a table with two columns that lists all your favorite colors and their hexadecimal equivalents. The background of each table row should be the same as the color named in the row as shown in the screenshot below.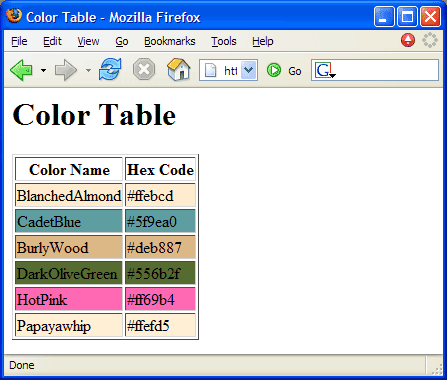
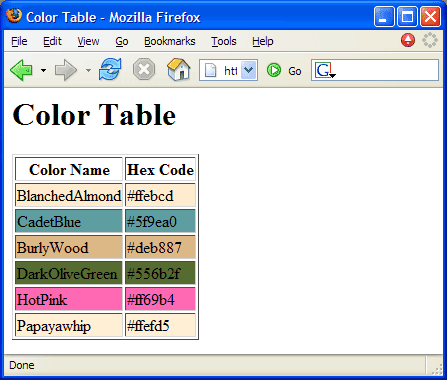
- Open Arrays/Exercises/ColorTable2.php for editing.
- Create an associative array that holds color hex codes indexed by their color names, which can be found at https://www.w3schools.com/colors/colors_names.asp.
- After the existing table row, write code to loop through the array outputting a table row with two columns for each element in the array.
- Test your solution in a browser.
Solution:
Arrays/Solutions/ColorTable2.php
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title>Color Table</title> </head> <body> <h1>Color Table</h1> <?php $favColors = array(); $favColors['BlanchedAlmond'] = '#ffebcd'; $favColors['CadetBlue'] = '#5f9ea0'; $favColors['BurlyWood'] = '#deb887'; $favColors['DarkOliveGreen'] = '#556b2f'; $favColors['HotPink'] = '#ff69b4'; $favColors['Papayawhip'] = '#ffefd5'; ?> <table border="1"> <tr> <th>Color Name</th> <th>Hex Code</th> </tr> <?php foreach ($favColors as $key => $item) { echo " <tr bgcolor='$key'> <td>$key</td> <td>$item</td> </tr>"; } ?> </table> </body> </html>
Two-dimensional Arrays
In PHP, two-dimensional arrays are arrays that contain arrays. You can think of the outer array as containing the rows and the inner arrays as containing the data cells in those rows. For example, a two-dimensional array called
$rockBands
could contain the names of the bands and some of the songs that they sing. Below is a grid that represents such a two-dimensional array.rockBand | Song1 | Song2 | Song3 |
---|---|---|---|
Beatles | Love Me Do | Hey Jude | Helter Skelter |
Rolling Stones | Waiting on a Friend | Angie | Yesterday's Papers |
Eagles | Life in the Fast Lane | Hotel California | Best of My Love |
The following code creates this two-dimensional array. The internal arrays are highlighted. Note that the header row is not included.
Syntax
$rockBands = array( array('Beatles','Love Me Do', 'Hey Jude','Helter Skelter'), array('Rolling Stones','Waiting on a Friend','Angie', 'Yesterday\'s Papers'), array('Eagles','Life in the Fast Lane','Hotel California', 'Best of My Love') )
Reading from Two-dimensional Arrays
To read an element from a two-dimensional array, you must first identify the index of the "row" and then identify the index of the "column." For example, the song "Angie" is in row 1, column 2, so it is identified as
$rockBands[1][2]
.
Remember that the first row is row 0 and the first column is column 0.
Looping through Two-dimensional Arrays
To loop through a two-dimensional array, you need to nest one loop inside of another. The following code will create an HTML table from our two-dimensional array.
Syntax
<table border="1"> <?php foreach($rockBands as $rockBand) { echo "<tr>"; foreach($rockBand as $item) { echo "<td>$item</td>"; } echo "</tr>"; } ?> </table>
The above code snippets are combined in the following example to output a rockBands table.
Code Sample:
Arrays/Demos/TwoDimensionalArrays.php
<!DOCTYPE HTML> <html> <head> <meta charset="UTF-8"> <title>Two-dimensional Arrays</title> </head> <body> <h1>Two-Dimensional Arrays</h1> <?php $rockBands = array( array('Beatles','Love Me Do', 'Hey Jude','Helter Skelter'), array('Rolling Stones','Waiting on a Friend','Angie','Yesterday\'s Papers'), array('Eagles','Life in the Fast Lane','Hotel California','Best of My Love') ); ?> <table border="1"> <tr> <th>rockBand</th> <th>Song 1</th> <th>Song 2</th> <th>Song 3</th> </tr> <?php foreach($rockBands as $rockBand) { echo '<tr>'; foreach($rockBand as $item) { echo "<td>$item</td>"; } echo '</tr>'; } ?> </table> </body> </html>
Array Manipulation Functions
The following table shows some of the more common array manipulation functions.
For a complete list of array functions, see http://www.php.net/array
Function | Explanation |
---|---|
sort() | Sorts an array alphabetically. Elements will be assigned to new index numbers. |
asort() | Sorts associative arrays alphabetically by value. The index association remains intact. |
ksort() | Sorts associative arrays alphabetically by key. The index association remains intact. |
rsort() | Reverse sorts an array alphabetically. Elements will be assigned to new index numbers. |
arsort() | Reverse sorts associative arrays alphabetically by value. The index association remains intact. |
krsort() | Reverse sorts associative arrays alphabetically by key. The index association remains intact. |
shuffle() | Randomly sorts the array. |
array_reverse() | Returns an array with the elements in reverse order. |
array_walk() | Applies a user function to every element of an array. |
count() | Returns the number of elements in an array. |
explode() | Converts a string to an array by splitting it on a specified separator. |
is_array() | Takes one parameter and returns true or false depending on whether the parameter passed is an array. |
array_keys() | Returns all the keys of an associative array as an array. |
array_key_exists() | Checks to see if a specified key exists in an array. |
0 comments:
Post a Comment