Nowadays, the barcode is a common thing for any product tagged with its body. Barcode is used to store various kind of data such as product code, price, manufacturer details and so on. So, in this tutorial, I will show you how to generate barcode using PHP. The following example of PHP barcode generator is very easy to learn and implement. You may also like to read – How to generate QR code using PHP and Ajax.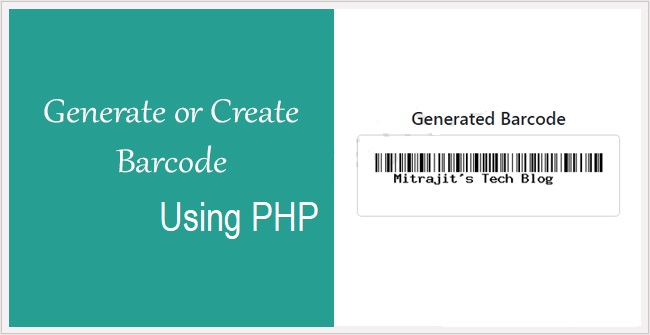
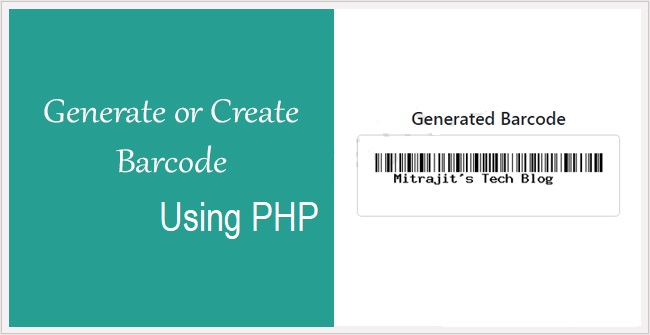
File Structure
Before proceeding, have a look at the file structure. Apart from bootstrap folder this example contains only two files –
- index.php
- barcode.php
Barcode Generator Library (barcode.php)
In order to generate barcode using PHP, you need to download a PHP library from Github and place it within your project folder. But you don’t need to do that because it is already included in the complete source code. Download it from the below Download link.
Different Parameters to Generate Barcode
- String: Whatever you want
- Code Type:
- Codabar
- Code128 (Default)
- Code2of5
- Code39
- Orientation:
- Horizontal (Default)
- Vertical
- Size:
- 10 (Minimum)
- 20 (Default)
- 400 (Maximum)
- Print:
- True (Display the string below the barcode)
- False
HTML – Barcode Generator Form (index.php)
- <div class="container-fluid">
- <div class="row">
- <div class="col-md-12">
- <h1 class="top-margin">Read the full article on -- <a href="http://www.mitrajit.com/generate-barcode-using-php/" target="_blank">How to generate barcode using PHP</a> in <a href="http://www.mitrajit.com/">Mitrajit's Tech Blog</a></h1>
- </div>
- </div>
- <form class="form-horizontal" method="POST" id="barcodeForm">
- <div class="row">
- <div class="col-md-12">
- <div class="form-group">
- <label>String</label>
- <input type="text" name="string" class="form-control" value="">
- </div>
- </div>
- </div>
- <div class="row">
- <div class="col-md-3">
- <div class="form-group">
- <label>Code Type</label>
- <select name="type" id="type" class="form-control">
- <option value="codebar">Codebar</option>
- <option value="code128" selected="selected">Code128</option>
- <option value="code2of5">Code2of5</option>
- <option value="code39">Code39</option>
- </select>
- </div>
- </div>
- <div class="col-md-3">
- <div class="form-group">
- <label>Orientation</label>
- <select name="orientation" class="form-control" required>
- <option value="horizontal" selected="selected">Horizontal</option>
- <option value="vertical">Vertical</option>
- </select>
- </div>
- </div>
- <div class="col-md-3">
- <div class="form-group">
- <label>Size</label>
- <input type="number" name="size" id="size" class="form-control" min="10" max="400" step="10" value="20" required>
- </div>
- </div>
- <div class="col-md-3">
- <div class="form-group">
- <label>Print</label>
- <select name="print" id="print" class="form-control" required>
- <option value="true" selected="selected">True</option>
- <option value="false">False</option>
- </select>
- </div>
- </div>
- </div>
- <div class="row text-center">
- <div class="col-md-12">
- <input type="submit" name="submit" class="btn btn-success text-center form-controll" id="" value="Generate Barcode">
- </div>
- </div>
- </form>
- </div>
The above HTML code for Barcode generator form is looking huge but don’t be afraid, it is simply a form using bootstrap.
PHP – Generator Barcode (index.php)
- <?php
- if(isset($_POST['submit'])) {
- $string = trim($_POST['string']);
- $type=$_POST['type'];
- $orientation=$_POST['orientation'];
- $size=$_POST['size'];
- $print=$_POST['print'];
- if($string != '') {
- echo '<h5>Generated Barcode</h5>';
- echo '<img class="barcode" alt="'.$string.'" src="barcode.php?text='.$string.'&codetype='.$type.'&orientation='.$orientation.'&size='.$size.'&print='.$print.'"/>';
- } else {
- echo 'Please enter a string!';
- }
- }
- ?>
Pass the form data as URL parameters to generate the barcode as an image.
This requires the use of barcode generate Asp.net technology, the author introduced in great detail
ReplyDelete